Java Strings Part Two- 16 Problems with Solutions
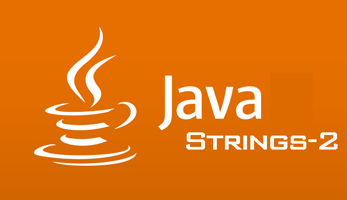
Problem-1 Given a string, return a string where for every char in the original, there are two chars. Example doubleChar(“The”) → “TThhee” doubleChar(“AAbb”) → “AAAAbbbb” doubleChar(“Hi-There”) → “HHii–TThheerree” Solution public String doubleChar(String str) { String result=””; for (int i=0;i<str.length();i++){ result+=str.substring(i,i+1)+str.substring(i,i+1); } return result; } Problem-2 Return the number of times that the string “hi” appears … [Read more…]